|
C Program To Implement Stack Data Structure Using Single Linked List: |
|
#include
#include
#include
#include
/* Node decleration */
struct node
{
int data;
struct node *link; //to maintain the link other nodes
};
struct node *top,*temp;
void create();
void push();
void pop();
void display();
/* create function create the head node */
void create()
{
printf("\nENTER THE FIRST ELEMENT: ");
top=(struct node *)malloc(sizeof(struct node));
scanf("%d",&top->data);
top->link=NULL;
temp=top;
}
/* display function visit the linked list from top to end */
void display()
{
top=temp; // bring the top to top position
printf("\n");
while(top!=NULL)
{
printf("%d\n",top->data);
top=top->link; // Now top points the previous node in the list
}
}
void push()
{
printf("\nENTER THE NEXT ELEMENT: ");
top=(struct node *)malloc(sizeof(struct node));
scanf("%d",&top->data);
top->link=temp;
temp=top;
}
void pop()
{
if(temp==NULL)
{
printf("\nSTACK IS EMPTY\n");
}
else
{
top=temp;
printf("\nDELETED ELEMENT IS %d\n",temp->data);
temp=temp->link;
free(top);
}
}
void main()
{
int ch;
clrscr();
while(1)
{
printf("\n\n 1.CREATE \n 2.PUSH \n 3.POP \n 4.EXIT \n");
printf("\n ENTER YOUR CHOICE : ");
scanf("%d",&ch);
switch(ch)
{
case 1:
create();
display();
break;
case 2:
push();
display();
break;
case 3:
pop();
display();
break;
case 4:
exit(0);
}
}
}
SAMPLE INPUT AND OUTPUT:
STACK
1. CREATE
2. PUSH
3. POP
4. EXIT
ENTER YOUR CHOICE : 1
ENTER THE FIRST ELEMENT : 10
10
STACK
1. CREATE
2. PUSH
3. POP
4. EXIT
ENTER YOUR CHOICE: 2
ENTER THE NEXT ELEMENT: 30
10
30
STACK
1. CREATE
2. PUSH
3. POP
4. EXIT
ENTER YOUR CHOICE: 3
DELETED ELEMENT IS 30
STACK
1. CREATE
2. PUSH
3. POP
4. EXIT
ENTER YOUR CHOICE: 3
DELETED ELEMENT IS 10
STACK
1. CREATE
2. PUSH
3. POP
4. EXIT
ENTER YOUR CHOICE: 3
STACK IS EMPTY.
|
|
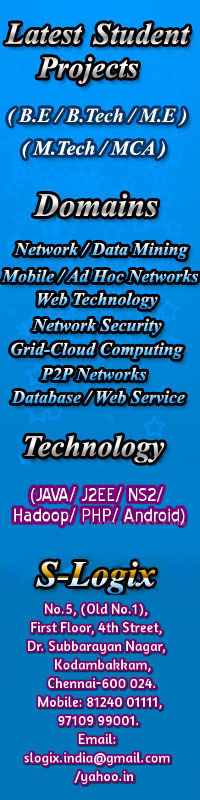
⇓ Student Projects ⇓
⇑ Student Projects ⇑
|