Banking System
Account Detail
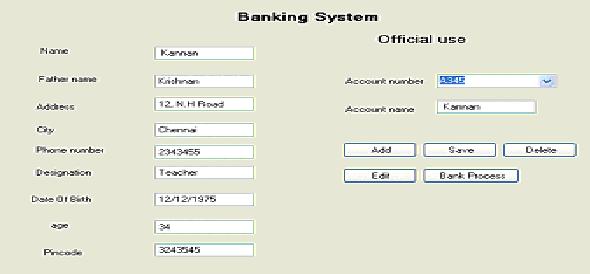
Saving
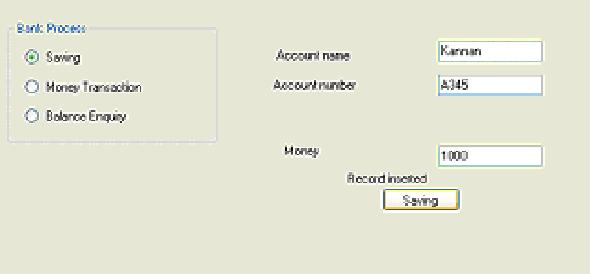
Money Transaction
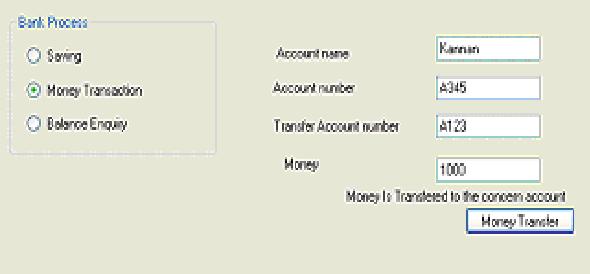
Balance Enquiry
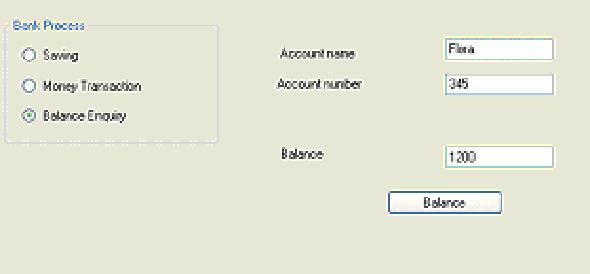
Coding:
Imports System.Data.SqlClient
Public Class Form1
Dim con As New SqlConnection
Dim cmd As New SqlCommand
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
ComboBox1.Visible = False
txtname.Text = " "
txtfname.Text = " "
txtadd.Text = " "
txtcity.Text = " "
txtpno.Text = " "
txtdes.Text = " "
txtacno.Text = " "
TextBox1.Text = " "
TextBox4.Text = " "
TextBox2.Text = " "
TextBox3.Text = " "
End Sub
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
ComboBox1.Visible = True
Label14.Visible = False
con = New SqlConnection("Data Source=COMPUTER10;Initial Catalog=banking;Integrated Security=TRUE")
Try
Dim cmd1 As New SqlCommand
Dim ad1 As New SqlDataAdapter
Dim d1 As New DataSet
cmd1 = New SqlCommand("select acno from newuser", con)
ad1 = New SqlDataAdapter(cmd1)
d1 = New DataSet
ad1.Fill(d1)
Dim i As Integer
For i = 0 To d1.Tables(0).Rows.Count - 1
ComboBox1.Items.Add(d1.Tables(0).Rows(i).Item(0))
Next
Catch ex As Exception
MsgBox(ex.Message)
End Try
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
Label14.Visible = True
con.Open()
cmd = New SqlCommand("insert into newuser values('" & txtname.Text & "','" & txtfname.Text & "','" & txtadd.Text & "','" & txtcity.Text & "','" & txtpno.Text & "','" & txtdes.Text & "','" & TextBox2.Text & "','" & TextBox1.Text & "','" & TextBox3.Text & "','" & txtacno.Text & "','" & TextBox4.Text & "')", con)
cmd.ExecuteNonQuery()
con.Close()
Label14.Text = "Record Inserted"
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
con.Open()
cmd = New SqlCommand("delete from newuser where acno='" & ComboBox1.SelectedItem & "'", con)
cmd.ExecuteNonQuery()
con.Close()
Label14.Visible = True
Label14.Text = "Record Deleted"
txtname.Text = " "
txtfname.Text = " "
txtadd.Text = " "
txtcity.Text = " "
txtpno.Text = " "
txtdes.Text = " "
txtacno.Text = " "
TextBox1.Text = " "
TextBox4.Text = " "
TextBox2.Text = " "
TextBox3.Text = " "
End Sub
Private Sub ComboBox1_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ComboBox1.SelectedIndexChanged
Try
con.Open()
Dim cmd2 As New SqlCommand
Dim ad2 As New SqlDataAdapter
Dim ds As New DataSet
cmd2 = New SqlCommand("select * from newuser where acno='" & ComboBox1.SelectedItem & "'", con)
ad2 = New SqlDataAdapter(cmd2)
ad2.Fill(ds)
If ds.Tables(0).Rows.Count > 0 Then
txtname.Text = ds.Tables(0).Rows(0).Item(0)
txtfname.Text = ds.Tables(0).Rows(0).Item(1)
txtadd.Text = ds.Tables(0).Rows(0).Item(2)
txtcity.Text = ds.Tables(0).Rows(0).Item(3)
txtpno.Text = ds.Tables(0).Rows(0).Item(4)
txtdes.Text = ds.Tables(0).Rows(0).Item(5)
txtacno.Text = ds.Tables(0).Rows(0).Item(6)
TextBox1.Text = ds.Tables(0).Rows(0).Item(7)
TextBox4.Text = ds.Tables(0).Rows(0).Item(0)
TextBox2.Text = ds.Tables(0).Rows(0).Item(6)
TextBox3.Text = ds.Tables(0).Rows(0).Item(8)
End If
con.Close()
Catch ex As Exception
MsgBox(ex.Message)
End Try
End Sub
Private Sub Button5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button5.Click
Form2.Show()
End Sub
End Class
Imports System.Data.SqlClient
Public Class Form2
Dim con As New SqlConnection
Private Sub Form2_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
con = New SqlConnection("Data Source=COMPUTER10;Initial Catalog=banking;Integrated Security=TRUE")
Label6.Visible = False
End Sub
Private Sub RadioButton1_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton1.CheckedChanged
Button2.Visible = False
Button3.Visible = False
Button1.Visible = True
Label4.Visible = False
Label5.Visible = True
Label3.Visible = False
TextBox3.Visible = False
End Sub
Private Sub RadioButton2_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton2.CheckedChanged
Button1.Visible = False
Button3.Visible = False
Button2.Visible = True
Label4.Visible = False
Label5.Visible = True
Label3.Visible = True
TextBox3.Visible = True
End Sub
Private Sub RadioButton3_CheckedChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles RadioButton3.CheckedChanged
Button1.Visible = False
Button2.Visible = False
Button3.Visible = True
Label4.Visible = True
Label5.Visible = False
Label3.Visible = False
TextBox3.Visible = False
End Sub
Private Sub Button3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button3.Click
Try
Dim cmd As New SqlCommand
Dim dr As SqlDataReader
con.Open()
cmd = New SqlCommand("select bal from account where acno = '" & TextBox2.Text & "'", con)
dr = cmd.ExecuteReader()
While dr.Read()
TextBox4.Text = dr(4)
End While
con.Close()
Catch ex As Exception
MsgBox(ex.Message)
End Try
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim cmd1 As New SqlCommand
Label6.Visible = True
con.Open()
cmd1 = New SqlCommand("insert into account values('" & TextBox2.Text & "','" & TextBox1.Text & "','" & TextBox4.Text & "')", con)
cmd1.ExecuteNonQuery()
con.Close()
Label6.Text = "Record inserted"
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
Label6.Visible = True
Label6.Text = "Money Is Transfered to the concern account"
End Sub
End Class
Database:
create database banking
create table newuser(name varchar(20),fname varchar(20),address varchar(20),city varchar(20),pno numeric,designation varchar(20),age numeric,dob varchar(20),accno numeric)
select * from newuser
create table account(name varchar(20), accno varchar(20), taccno numeric, money numeric, balance numeric)
|