Description:
This program is to play the video file in applet using Java Media Framework. In this program, we are giving the panel and bounds to display the file. This program uses Runnable and Controller Listener interfaces. Javax.media and javax.swing packages are included in this program. Using VedioAudioPlayer (String) method we are calling the init () method to initialize the player. After the start () method video file starts running.
Playing Video File in Java Applet:
import java.util.*;
import java.net.*;
import java.io.*;
import javax.media.*;
import javax.swing.*;
import java.awt.*;
public class Client2 extends javax.swing.JFrame implements ControllerListener,Runnable{
String filepath;
Player player;
Component visualComponent;
Component controlComponent;
Component progressBar;
boolean firstTime;
long CachingSize;
int controlPanelHeight;
int videoWidth;
int videoHeight;
/** Creates new form Client2 */
public Client2() {
System.out.println("Inside the Client2 Constructor");
initComponents();
setBounds(50,50,880,600);
}
public void run(){
System.out.println(" Inside the Run mehtod");
while(true){
}
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
private void initComponents() {//GEN-BEGIN:initComponents
jPanel1 = new javax.swing.JPanel();
getFile = new javax.swing.JButton();
panel = new java.awt.Panel();
getContentPane().setLayout(null);
setTitle("Click the Button to play Video File");
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
exitForm(evt);
}
});
jPanel1.setLayout(null);
jPanel1.setBackground(new java.awt.Color(123, 153, 153));
getFile.setBackground(new java.awt.Color(204, 255, 204));
getFile.setForeground(new java.awt.Color(51, 51, 255));
getFile.setText("PlayFile");
getFile.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
getFileActionPerformed(evt);
}
});
jPanel1.add(getFile);
getFile.setBounds(260, 130, 72, 26);
panel.setLayout(null);
panel.setName("panel");
jPanel1.add(panel);
panel.setBounds(440, 100, 340, 280);
getContentPane().add(jPanel1);
jPanel1.setBounds(0, 0, 880, 580);
pack();
}//GEN-END:initComponents
private void FilesActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_FilesActionPerformed
}//GEN-LAST:event_FilesActionPerformed
private void peersActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_peersActionPerformed
}//GEN-LAST:event_peersActionPerformed
private void ExitButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_ExitButtonActionPerformed
System.exit(0);
}//GEN-LAST:event_ExitButtonActionPerformed
private void getFileActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_getFileActionPerformed
VedioAudioPlayer("C:\\P2PProxyfinal\\Client2\\TCache\\Temp.mpg");
}//GEN-LAST:event_getFileActionPerformed
/** Exit the Application */
private void exitForm(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_exitForm
}//GEN-LAST:event_exitForm
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
System.out.println("Client2 is Ready to Start");
new Client2().show();
}
public void VedioAudioPlayer(String file) {
System.out.println("The Asking File is ready to Play");
player = null;
visualComponent = null;
controlComponent = null;
progressBar = null;
firstTime = true;
CachingSize = 0L;
controlPanelHeight = 0;
videoWidth = 0;
videoHeight = 0;
filepath=file;
init();
}
public void init() {
String s = null;
MediaLocator medialocator = null;
Object obj = null;
URL url;
if(filepath == null)
Fatal("Invalid media file parameter");
try {
//url = new URL(filepath);
if((medialocator = new MediaLocator("file:"+filepath)) == null)
Fatal("Can't build URL for " + filepath);
try {
player = Manager.createPlayer(medialocator);
player.start();
player.addControllerListener(this);
player.realize();
}
catch(NoPlayerException noplayerexception) {
System.out.println(noplayerexception);
Fatal("Could not create player for " + medialocator);
}
}
catch(MalformedURLException _ex) {
Fatal("Invalid media file URL!");
}
catch(IOException _ex) {
Fatal("IO exception creating player for " + medialocator);
}
}
public synchronized void controllerUpdate(ControllerEvent controllerevent) {
if(player == null)
return;
if(controllerevent instanceof RealizeCompleteEvent) {
if(progressBar != null) {
panel.remove(progressBar);
progressBar = null;
}
int i = 320;
int j = 0;
if(controlComponent == null && (controlComponent = player.getControlPanelComponent()) != null) {
controlPanelHeight = controlComponent.getPreferredSize().height;
panel.add(controlComponent);
j += controlPanelHeight;
}
if(visualComponent == null && (visualComponent = player.getVisualComponent()) != null) {
panel.add(visualComponent);
Dimension dimension = visualComponent.getPreferredSize();
videoWidth = dimension.width;
videoHeight = dimension.height;
i = videoWidth;
j += videoHeight;
visualComponent.setBounds(0, 0, videoWidth, videoHeight);
}
//panel.setBounds(0, 0, i, j);
if(controlComponent != null) {
controlComponent.setBounds(0, videoHeight, i, controlPanelHeight);
controlComponent.invalidate();
}
} else
if(controllerevent instanceof CachingControlEvent) {
if(player.getState() > 200)
return;
CachingControlEvent cachingcontrolevent = (CachingControlEvent)controllerevent;
CachingControl cachingcontrol = cachingcontrolevent.getCachingControl();
if(progressBar == null && (progressBar = cachingcontrol.getControlComponent()) != null) {
panel.add(progressBar);
panel.setSize(progressBar.getPreferredSize());
validate();
}
} else
if(controllerevent instanceof EndOfMediaEvent) {
player.setMediaTime(new Time(0L));
player.stop();
} else
if(controllerevent instanceof ControllerErrorEvent) {
player = null;
Fatal(((ControllerErrorEvent)controllerevent).getMessage());
} else
if(controllerevent instanceof ControllerClosedEvent)
panel.removeAll();
}
void Fatal(String s) {
System.err.println("FATAL ERROR: " + s);
throw new Error(s);
}
public void destroy() {
if(player != null)
player.close();
}
public void start() {
if(player != null)
player.start();
}
public void stop() {
if(player != null) {
player.stop();
player.deallocate();
}
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton getFile;
private javax.swing.JLabel jLabel2;
private javax.swing.JPanel jPanel1;
public java.awt.Panel panel;
// End of variables declaration//GEN-END:variables
}
Sample ScreenShot:
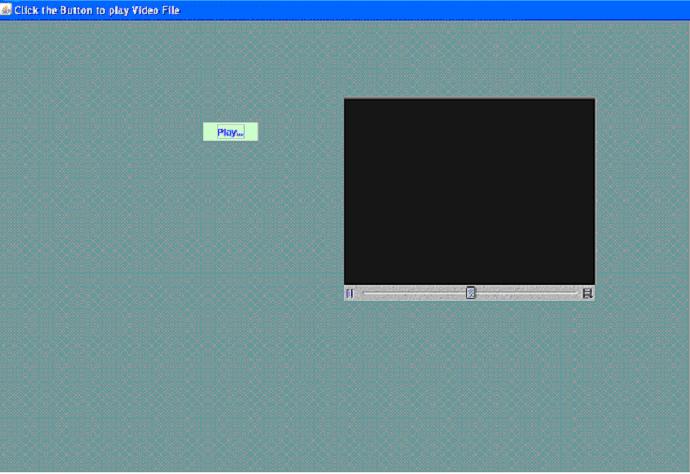
|