Description:
This program is to verify the userid and password of the user. Client sends the data to the server for verification. To protect these details from the hackers MD5 and RSA algorithms are used. In client side User’s password is encrypted using RSA. For this encrypted message hashcode values are generated using MD5 algorithm. Both encrypted message and hashcode value are sent to the server. Server also generates the hashcode for the received message. Generated hashcode is verified with the received hashcode, if both are equal then the message is decrypted and checked with the userid password on the database. Otherwise server asks the client to resend the message. Encryption, Decryption classes are used in message integrity. If any changes are made in the encrypted message then the same hashcode will not be generated.
Login form Validation with MD5 for message integrity Using Java :
/*ClientLogin*/
import javax.swing.*;
import java.io.*;
import java.net.*;
import java.util.*;
import java.math.*;
public class ClientLogin extends javax.swing.JFrame {
public ClientLogin() {
initComponents();
userInitComponents();
}
public void userInitComponents(){
setBounds(0,0,800,600);
}
private void initComponents() {//GEN-BEGIN:initComponents
panel_container = new javax.swing.JPanel();
panel_login_con = new javax.swing.JPanel();
l_id = new javax.swing.JLabel();
_pass = new javax.swing.JLabel();
b_login = new javax.swing.JButton();
b_clear = new javax.swing.JButton();
t_id = new javax.swing.JTextField();
t_pass = new javax.swing.JPasswordField();
getContentPane().setLayout(null);
setTitle("Client Login");
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
exitForm(evt);
}
});
panel_container.setLayout(null);
panel_container.setBackground(new java.awt.Color(153, 153, 255));
panel_container.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
panel_login_con.setLayout(null);
panel_login_con.setBackground(new java.awt.Color(153, 153, 255));
panel_login_con.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
l_id.setFont(new java.awt.Font("Times New Roman", 1, 18));
l_id.setForeground(new java.awt.Color(255, 0, 0));
l_id.setText("CLIENT ID");
panel_login_con.add(l_id);
l_id.setBounds(60, 20, 130, 30);
_pass.setFont(new java.awt.Font("Times New Roman", 1, 18));
_pass.setForeground(new java.awt.Color(255, 0, 0));
_pass.setText("PASSWORD");
panel_login_con.add(_pass);
_pass.setBounds(60, 80, 130, 30);
b_login.setBackground(new java.awt.Color(153, 153, 255));
b_login.setFont(new java.awt.Font("Times New Roman", 1, 18));
b_login.setForeground(new java.awt.Color(255, 0, 0));
b_login.setText("LOGIN");
b_login.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
b_login.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
b_loginActionPerformed(evt);
}
});
panel_login_con.add(b_login);
b_login.setBounds(140, 150, 90, 30);
b_clear.setBackground(new java.awt.Color(153, 153, 255));
b_clear.setFont(new java.awt.Font("Times New Roman", 1, 18));
b_clear.setForeground(new java.awt.Color(255, 0, 0));
b_clear.setText("CLEAR");
b_clear.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
b_clear.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
b_clearActionPerformed(evt);
}
});
panel_login_con.add(b_clear);
b_clear.setBounds(290, 150, 90, 30);
t_id.setBackground(new java.awt.Color(255, 255, 255));
t_id.setFont(new java.awt.Font("Times New Roman", 1, 14));
t_id.setForeground(new java.awt.Color(51, 51, 255));
t_id.setText(" ");
t_id.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.LOWERED));
panel_login_con.add(t_id);
t_id.setBounds(200, 20, 180, 30);
t_pass.setFont(new java.awt.Font("Times New Roman", 1, 14));
t_pass.setForeground(new java.awt.Color(0, 51, 255));
t_pass.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.LOWERED));
panel_login_con.add(t_pass);
t_pass.setBounds(200, 80, 180, 30);
panel_container.add(panel_login_con);
panel_login_con.setBounds(150, 160, 470, 220);
getContentPane().add(panel_container);
panel_container.setBounds(0, 0, 800, 600);
pack();
}//GEN-END:initComponents
private void b_loginActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_b_loginActionPerformed
boolean validdata=true;
String userid=t_id.getText().trim();
String password=t_pass.getText().trim();
if(userid.equals("")||password.equals("")){
validdata=false;
JOptionPane.showMessageDialog(this,"Please Enter ID and Password","Login error Message",2);
}
if(validdata){
String server_result=loginValidation(userid,password);
if(server_result.equalsIgnoreCase("invalid")){
JOptionPane.showMessageDialog(this,"Invalid User ID and Password","Login error Message",2);
}
else if(server_result.equalsIgnoreCase("wrongpass")){
JOptionPane.showMessageDialog(this,"Wrong sword","Login error Message",2);
}
else if(server_result.equalsIgnoreCase("valid")){
//move to next form
//valid login
setVisible(false);
//ClientForm client=new ClientForm(userid);
//client.setVisible(true);
JOptionPane.showMessageDialog(this,"Authentication Success");
}
}
}//GEN-LAST:event_b_loginActionPerformed
private void b_clearActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_b_clearActionPerformed
t_id.setText("");
t_pass.setText("");
}//GEN-LAST:event_b_clearActionPerformed
private void exitForm(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_exitForm
System.exit(0);
}//GEN-LAST:event_exitForm
public static void main(String args[]) {
new ClientLogin().show();
}
public String loginValidation(String userid,String password){
String server_result="";
String login_result="";
String nhash;
BigInteger[] ciphertext = null;
BigInteger n = null;
BigInteger d = null;
Vector send_packet=new Vector();
send_packet.add("login");
send_packet.add(userid);
RSA rsa = new RSA( 8 ) ;
n=rsa.getN();
d=rsa.getD();
ciphertext = rsa.encrypt(password ) ;
System.out.println("Client side ");
//System.out.println("Encrypted value of the password"+ciphertext);
StringBuffer bf = new StringBuffer();
for( int i = 0 ; i < ciphertext.length ; i++ )
{
bf.append( ciphertext[i].toString( 16 ).toUpperCase() ) ;
if( i != ciphertext.length - 1 )
System.out.print( " " ) ;
}
String message=bf.toString();
System.out.println("Encrypted Message"+message);
byte buf[] = message.getBytes();
MD5 md = new MD5();
byte out[] = new byte[16];
md.update(buf);
md.md5final(out);
nhash = md.dumpBytes(out);
System.out.println("Hashcode"+nhash);
send_packet.add(nhash);
send_packet.add(ciphertext);
send_packet.add(n);
send_packet.add(d);
try{
System.out.println("Client Communicate with server...");
Socket server=new Socket("localhost",4444);
ObjectOutputStream toserver=new ObjectOutputStream(server.getOutputStream());
toserver.writeObject(send_packet);
System.out.println("Client Sending data.....");
ObjectInputStream fromserver=new ObjectInputStream(server.getInputStream());
Vector recived_packet=(Vector)fromserver.readObject();
login_result=(String)recived_packet.get(0);
System.out.println("Client Recived data.....");
toserver.flush();
toserver.close();
fromserver.close();
}
catch(Exception e){
e.printStackTrace();
}
if(login_result.equalsIgnoreCase("valid")){
server_result="valid";
}
else if(login_result.equalsIgnoreCase("invalid")){
server_result="invalid";
}
else if(login_result.equalsIgnoreCase("wrongpass")){
server_result="wrongpass";
}
System.out.println("Server Result :"+server_result);
return server_result;
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JPasswordField t_pass;
private javax.swing.JPanel panel_login_con;
private javax.swing.JButton b_clear;
private javax.swing.JLabel _pass;
private javax.swing.JLabel l_id;
private javax.swing.JButton b_login;
private javax.swing.JTextField t_id;
private javax.swing.JPanel panel_container;
// End of variables declaration//GEN-END:variables
}
/*Decryption*/
import java.io.*;
import java.util.*;
import java.math.*;
public class Decryption{
BigInteger n = null;
BigInteger d = null;
BigInteger [] enc = null;
String dhash=null;
String rhash=null;
Vector vec=new Vector();
public String dataIntegrity(Vector vect){
vec=vect;
rhash=(String)vec.elementAt(0);
enc =(BigInteger[]) vec.elementAt(1);
StringBuffer bf = new StringBuffer();
for( int i = 0 ; i < enc.length ; i++ ){
bf.append( enc[i].toString( 16 ).toUpperCase() ) ;
if( i != enc.length - 1 )
System.out.print( " " ) ;
}
String message=bf.toString();
//System.out.println("Message"+message);
n = (BigInteger)vec.elementAt(2);
d = (BigInteger)vec.elementAt(3);
//in.close();
byte buf[] = message.getBytes();
MD5 md = new MD5();
byte out[] = new byte[16];
md.update(buf);
md.md5final(out);
String nhash = md.dumpBytes(out);
//sha256New sha = new sha256New();
//String nhash = sha.hash(message.getBytes());
System.out.println("hash: "+nhash);
if(rhash.equals(nhash))
{
RSA rsa = new RSA( 8 ) ;
dhash = rsa.decrypt( enc ,d,n) ;
//System.out.println("Correct message"+dhash);
}
return dhash;
}
}
/*Encryption*/
import java.io.*;
import java.util.*;
import java.math.*;
public class Encryption{
public String Data;
String nhash;
BigInteger[] ciphertext = null;
BigInteger n = null;
BigInteger d = null;
Vector v=new Vector();
public Vector dataIntegrity(String Original){
Data=Original;
RSA rsa = new RSA( 8 ) ;
n=rsa.getN();
d=rsa.getD();
ciphertext = rsa.encrypt(Data ) ;
StringBuffer bf = new StringBuffer();
for( int i = 0 ; i < ciphertext.length ; i++ )
{
bf.append( ciphertext[i].toString( 16 ).toUpperCase() ) ;
if( i != ciphertext.length - 1 )
System.out.print( " " ) ;
}
String message=bf.toString();
//System.out.println("Message"+message);
byte buf[] = message.getBytes();
MD5 md = new MD5();
byte out[] = new byte[16];
md.update(buf);
md.md5final(out);
nhash = md.dumpBytes(out);
//System.out.println("hash: "+nhash);
v.addElement(nhash);
v.addElement(ciphertext);
v.addElement(n);
v.addElement(d);
return v;
}
}
/*MD5.java*/
import java.io.*;
import java.net.*;
import java.util.*;
import java.math.*;
public class MD5 {
int buf[]; // These were originally unsigned ints.
// This Java code makes an effort to avoid sign traps.
// buf[] is where the hash accumulates.
long bits; // This is the count of bits hashed so far.
byte in[]; // This is a buffer where we stash bytes until we have
// enough (64) to perform a transform operation.
int inint[];
// inint[] used and discarded inside transform(),
// but why allocate it over and over?
// (In the C version this is allocated on the stack.)
public MD5() {
buf = new int[4];
// fill the hash accumulator with a seed value
buf[0] = 0x67452301;
buf[1] = 0xefcdab89;
buf[2] = 0x98badcfe;
buf[3] = 0x10325476;
// initially, we've hashed zero bits
bits = 0L;
in = new byte[64];
inint = new int[16];
}
public void update(byte[] newbuf) {
update(newbuf, 0, newbuf.length);
}
public void update(byte[] newbuf, int length) {
update(newbuf, 0, length);
}
public void update(byte[] newbuf, int bufstart, int buflen) {
int t;
int len = buflen;
// shash old bits value for the "Bytes already in" computation
// just below.
t = (int) bits; // (int) cast should just drop high bits, I hope
/* update bitcount */
/* the C code used two 32-bit ints separately, and carefully
* ensured that the carry carried.
* Java has a 64-bit long, which is just what the code really wants.
*/
bits += (long)(len<<3);
t = (t >>> 3) & 0x3f; /* Bytes already in this->in */
/* Handle any leading odd-sized chunks */
/* (that is, any left-over chunk left by last update() */
if (t!=0) {
int p = t;
t = 64 - t;
if (len < t) {
System.arraycopy(newbuf, bufstart, in, p, len);
return;
}
System.arraycopy(newbuf, bufstart, in, p, t);
transform();
bufstart += t;
len -= t;
}
/* Process data in 64-byte chunks */
while (len >= 64) {
System.arraycopy(newbuf, bufstart, in, 0, 64);
transform();
bufstart += 64;
len -= 64;
}
/* Handle any remaining bytes of data. */
/* that is, stash them for the next update(). */
System.arraycopy(newbuf, bufstart, in, 0, len);
}
/*
* Final wrapup - pad to 64-byte boundary with the bit pattern
* 1 0* (64-bit count of bits processed, MSB-first)
*/
public void md5final(byte[] digest) {
/* "final" is a poor method name in Java. :v) */
int count;
int p; // in original code, this is a pointer; in this java code
// it's an index into the array this->in.
/* Compute number of bytes mod 64 */
count = (int) ((bits >>> 3) & 0x3F);
/* Set the first char of padding to 0x80. This is safe since there is
always at least one byte free */
p = count;
in[p++] = (byte) 0x80;
/* Bytes of padding needed to make 64 bytes */
count = 64 - 1 - count;
/* Pad out to 56 mod 64 */
if (count < 8) {
/* Two lots of padding: Pad the first block to 64 bytes */
zeroByteArray(in, p, count);
transform();
/* Now fill the next block with 56 bytes */
zeroByteArray(in, 0, 56);
} else {
/* Pad block to 56 bytes */
zeroByteArray(in, p, count - 8);
}
/* Append length in bits and transform */
// Could use a PUT_64BIT... func here. This is a fairly
// direct translation from the C code, where bits was an array
// of two 32-bit ints.
int lowbits = (int) bits;
int highbits = (int) (bits >>> 32);
PUT_32BIT_LSB_FIRST(in, 56, lowbits);
PUT_32BIT_LSB_FIRST(in, 60, highbits);
transform();
PUT_32BIT_LSB_FIRST(digest, 0, buf[0]);
PUT_32BIT_LSB_FIRST(digest, 4, buf[1]);
PUT_32BIT_LSB_FIRST(digest, 8, buf[2]);
PUT_32BIT_LSB_FIRST(digest, 12, buf[3]);
/* zero sensitive data */
/* notice this misses any sneaking out on the stack. The C
* version uses registers in some spots, perhaps because
* they care about this.
*/
zeroByteArray(in);
zeroIntArray(buf);
bits = 0;
zeroIntArray(inint);
}
public static void main(String args[]) {
// This main() method was created to easily test
// this class. It hashes whatever's on System.in.
byte buf[] = new byte[397];
// arbitrary buffer length designed to irritate update()
int rc;
MD5 md = new MD5();
byte out[] = new byte[16];
int i;
int len = 0;
try {
while ((rc = System.in.read(buf, 0, 397)) > 0) {
md.update(buf, rc);
len += rc;
}
} catch (IOException ex) {
ex.printStackTrace();
return;
}
md.md5final(out);
System.out.println("file length: "+len);
System.out.println("hash: "+dumpBytes(out));
}
/////////////////////////////////////////////////////////////////////
// Below here ye will only finde private functions //
/////////////////////////////////////////////////////////////////////
// There must be a way to do these functions that's
// built into Java, and I just haven't noticed it yet.
private void zeroByteArray(byte[] a) {
zeroByteArray(a, 0, a.length);
}
private void zeroByteArray(byte[] a, int start, int length) {
setByteArray(a, (byte) 0, start, length);
}
private void setByteArray(byte[] a, byte val, int start, int length) {
int i;
int end = start+length;
for (i=start; i<>>(32-s);
w += x;
return w;
}
private void transform() {
/* load in[] byte array into an internal int array */
int i;
int[] inint = new int[16];
for (i=0; i<16; i++) {
inint[i] = GET_32BIT_LSB_FIRST(in, 4*i);
}
int a, b, c, d;
a = buf[0];
b = buf[1];
c = buf[2];
d = buf[3];
a = MD5STEP(F1, a, b, c, d, inint[0] + 0xd76aa478, 7);
d = MD5STEP(F1, d, a, b, c, inint[1] + 0xe8c7b756, 12);
c = MD5STEP(F1, c, d, a, b, inint[2] + 0x242070db, 17);
b = MD5STEP(F1, b, c, d, a, inint[3] + 0xc1bdceee, 22);
a = MD5STEP(F1, a, b, c, d, inint[4] + 0xf57c0faf, 7);
d = MD5STEP(F1, d, a, b, c, inint[5] + 0x4787c62a, 12);
c = MD5STEP(F1, c, d, a, b, inint[6] + 0xa8304613, 17);
b = MD5STEP(F1, b, c, d, a, inint[7] + 0xfd469501, 22);
a = MD5STEP(F1, a, b, c, d, inint[8] + 0x698098d8, 7);
d = MD5STEP(F1, d, a, b, c, inint[9] + 0x8b44f7af, 12);
c = MD5STEP(F1, c, d, a, b, inint[10] + 0xffff5bb1, 17);
b = MD5STEP(F1, b, c, d, a, inint[11] + 0x895cd7be, 22);
a = MD5STEP(F1, a, b, c, d, inint[12] + 0x6b901122, 7);
d = MD5STEP(F1, d, a, b, c, inint[13] + 0xfd987193, 12);
c = MD5STEP(F1, c, d, a, b, inint[14] + 0xa679438e, 17);
b = MD5STEP(F1, b, c, d, a, inint[15] + 0x49b40821, 22);
a = MD5STEP(F2, a, b, c, d, inint[1] + 0xf61e2562, 5);
d = MD5STEP(F2, d, a, b, c, inint[6] + 0xc040b340, 9);
c = MD5STEP(F2, c, d, a, b, inint[11] + 0x265e5a51, 14);
b = MD5STEP(F2, b, c, d, a, inint[0] + 0xe9b6c7aa, 20);
a = MD5STEP(F2, a, b, c, d, inint[5] + 0xd62f105d, 5);
d = MD5STEP(F2, d, a, b, c, inint[10] + 0x02441453, 9);
c = MD5STEP(F2, c, d, a, b, inint[15] + 0xd8a1e681, 14);
b = MD5STEP(F2, b, c, d, a, inint[4] + 0xe7d3fbc8, 20);
a = MD5STEP(F2, a, b, c, d, inint[9] + 0x21e1cde6, 5);
d = MD5STEP(F2, d, a, b, c, inint[14] + 0xc33707d6, 9);
c = MD5STEP(F2, c, d, a, b, inint[3] + 0xf4d50d87, 14);
b = MD5STEP(F2, b, c, d, a, inint[8] + 0x455a14ed, 20);
a = MD5STEP(F2, a, b, c, d, inint[13] + 0xa9e3e905, 5);
d = MD5STEP(F2, d, a, b, c, inint[2] + 0xfcefa3f8, 9);
c = MD5STEP(F2, c, d, a, b, inint[7] + 0x676f02d9, 14);
b = MD5STEP(F2, b, c, d, a, inint[12] + 0x8d2a4c8a, 20);
a = MD5STEP(F3, a, b, c, d, inint[5] + 0xfffa3942, 4);
d = MD5STEP(F3, d, a, b, c, inint[8] + 0x8771f681, 11);
c = MD5STEP(F3, c, d, a, b, inint[11] + 0x6d9d6122, 16);
b = MD5STEP(F3, b, c, d, a, inint[14] + 0xfde5380c, 23);
a = MD5STEP(F3, a, b, c, d, inint[1] + 0xa4beea44, 4);
d = MD5STEP(F3, d, a, b, c, inint[4] + 0x4bdecfa9, 11);
c = MD5STEP(F3, c, d, a, b, inint[7] + 0xf6bb4b60, 16);
b = MD5STEP(F3, b, c, d, a, inint[10] + 0xbebfbc70, 23);
a = MD5STEP(F3, a, b, c, d, inint[13] + 0x289b7ec6, 4);
d = MD5STEP(F3, d, a, b, c, inint[0] + 0xeaa127fa, 11);
c = MD5STEP(F3, c, d, a, b, inint[3] + 0xd4ef3085, 16);
b = MD5STEP(F3, b, c, d, a, inint[6] + 0x04881d05, 23);
a = MD5STEP(F3, a, b, c, d, inint[9] + 0xd9d4d039, 4);
d = MD5STEP(F3, d, a, b, c, inint[12] + 0xe6db99e5, 11);
c = MD5STEP(F3, c, d, a, b, inint[15] + 0x1fa27cf8, 16);
b = MD5STEP(F3, b, c, d, a, inint[2] + 0xc4ac5665, 23);
a = MD5STEP(F4, a, b, c, d, inint[0] + 0xf4292244, 6);
d = MD5STEP(F4, d, a, b, c, inint[7] + 0x432aff97, 10);
c = MD5STEP(F4, c, d, a, b, inint[14] + 0xab9423a7, 15);
b = MD5STEP(F4, b, c, d, a, inint[5] + 0xfc93a039, 21);
a = MD5STEP(F4, a, b, c, d, inint[12] + 0x655b59c3, 6);
d = MD5STEP(F4, d, a, b, c, inint[3] + 0x8f0ccc92, 10);
c = MD5STEP(F4, c, d, a, b, inint[10] + 0xffeff47d, 15);
b = MD5STEP(F4, b, c, d, a, inint[1] + 0x85845dd1, 21);
a = MD5STEP(F4, a, b, c, d, inint[8] + 0x6fa87e4f, 6);
d = MD5STEP(F4, d, a, b, c, inint[15] + 0xfe2ce6e0, 10);
c = MD5STEP(F4, c, d, a, b, inint[6] + 0xa3014314, 15);
b = MD5STEP(F4, b, c, d, a, inint[13] + 0x4e0811a1, 21);
a = MD5STEP(F4, a, b, c, d, inint[4] + 0xf7537e82, 6);
d = MD5STEP(F4, d, a, b, c, inint[11] + 0xbd3af235, 10);
c = MD5STEP(F4, c, d, a, b, inint[2] + 0x2ad7d2bb, 15);
b = MD5STEP(F4, b, c, d, a, inint[9] + 0xeb86d391, 21);
buf[0] += a;
buf[1] += b;
buf[2] += c;
buf[3] += d;
}
private int GET_32BIT_LSB_FIRST(byte[] b, int off) {
return
((int)(b[off+0]&0xff)) |
((int)(b[off+1]&0xff) << 8) |
((int)(b[off+2]&0xff) << 16) |
((int)(b[off+3]&0xff) << 24);
}
private void PUT_32BIT_LSB_FIRST(byte[] b, int off, int value) {
b[off+0] = (byte) (value & 0xff);
b[off+1] = (byte) ((value >> 8) & 0xff);
b[off+2] = (byte) ((value >> 16)& 0xff);
b[off+3] = (byte) ((value >> 24)& 0xff);
}
// These are debug routines I was using while trying to
// get this code to generate the same hashes as the C version.
// (IIRC, all the errors were due to the absence of unsigned
// ints in Java.)
/*
private void debugStatus(String m) {
System.out.println(m+":");
System.out.println("in: "+dumpBytes(in));
System.out.println("bits: "+bits);
System.out.println("buf: "
+Integer.toHexString(buf[0])+" "
+Integer.toHexString(buf[1])+" "
+Integer.toHexString(buf[2])+" "
+Integer.toHexString(buf[3]));
}
*/
public static String dumpBytes(byte[] bytes) {
int i;
StringBuffer sb = new StringBuffer();
for (i=0; i< 2) {
s = "0"+s;
}
if (s.length() > 2) {
s = s.substring(s.length()-2);
}
sb.append(s);
}
return sb.toString();
}
}
/*RSA*/
import java.math.BigInteger ;
import java.util.Random ;
import java.io.* ;
public class RSA
{
/**
* Bit length of each prime number.
*/
int primeSize ;
/**
* Two distinct large prime numbers p and q.
*/
BigInteger p, q ;
/**
* Modulus N.
*/
BigInteger N ;
/**
* r = ( p - 1 ) * ( q - 1 )
*/
BigInteger r ;
/**
* Public exponent E and Private exponent D
*/
BigInteger E, D ;
/**
* Constructor.
*
* @param primeSize Bit length of each prime number.
*/
public RSA( int primeSize )
{
this.primeSize = primeSize ;
// Generate two distinct large prime numbers p and q.
generatePrimeNumbers() ;
// Generate Public and Private Keys.
generatePublicPrivateKeys() ;
}
/**
* Generate two distinct large prime numbers p and q.
*/
public void generatePrimeNumbers()
{
p = new BigInteger( primeSize, 10, new Random() ) ;
do
{
q = new BigInteger( primeSize, 10, new Random() ) ;
}
while( q.compareTo( p ) == 0 ) ;
}
/**
* Generate Public and Private Keys.
*/
public void generatePublicPrivateKeys()
{
// N = p * q
N = p.multiply( q ) ;
// r = ( p - 1 ) * ( q - 1 )
r = p.subtract( BigInteger.valueOf( 1 ) ) ;
r = r.multiply( q.subtract( BigInteger.valueOf( 1 ) ) ) ;
// Choose E, coprime to and less than r
do
{
E = new BigInteger( 2 * primeSize, new Random() ) ;
}
while( ( E.compareTo( r ) != -1 ) || ( E.gcd( r ).compareTo( BigInteger.valueOf( 1 ) ) != 0 ) ) ;
// Compute D, the inverse of E mod r
D = E.modInverse( r ) ;
}
/**
* Encrypts the plaintext (Using Public Key).
*
* @param message String containing the plaintext message to be encrypted.
* @return The ciphertext as a BigInteger array.
*/
public BigInteger[] encrypt( String message )
{
int i ;
byte[] temp = new byte[1] ;
byte[] digits = message.getBytes() ;
BigInteger[] bigdigits = new BigInteger[digits.length] ;
for( i = 0 ; i < bigdigits.length ; i++ )
{
temp[0] = digits[i] ;
bigdigits[i] = new BigInteger( temp ) ;
}
BigInteger[] encrypted = new BigInteger[bigdigits.length] ;
for( i = 0 ; i < bigdigits.length ; i++ )
encrypted[i] = bigdigits[i].modPow( E, N ) ;
return( encrypted ) ;
}
/**
* Decrypts the ciphertext (Using Private Key).
*
* @param encrypted BigInteger array containing the ciphertext to be decrypted.
* @return The decrypted plaintext.
*/
public String decrypt( BigInteger[] encrypted,BigInteger D,BigInteger N )
{
int i ;
BigInteger[] decrypted = new BigInteger[encrypted.length] ;
for( i = 0 ; i < decrypted.length ; i++ )
decrypted[i] = encrypted[i].modPow( D, N ) ;
char[] charArray = new char[decrypted.length] ;
for( i = 0 ; i < charArray.length ; i++ )
charArray[i] = (char) ( decrypted[i].intValue() ) ;
return( new String( charArray ) ) ;
}
/**
* Get prime number p.
*
* @return Prime number p.
*/
public BigInteger getp()
{
return( p ) ;
}
/**
* Get prime number q.
*
* @return Prime number q.
*/
public BigInteger getq()
{
return( q ) ;
}
/**
* Get r.
*
* @return r.
*/
public BigInteger getr()
{
return( r ) ;
}
/**
* Get modulus N.
*
* @return Modulus N.
*/
public BigInteger getN()
{
return( N ) ;
}
/**
* Get Public exponent E.
*
* @return Public exponent E.
*/
public BigInteger getE()
{
return( E ) ;
}
/**
* Get Private exponent D.
*
* @return Private exponent D.
*/
public BigInteger getD()
{
return( D ) ;
}
/**
* RSA Main program for Unit Testing.
*/
public static void main( String[] args ) throws IOException
{
if( args.length != 1 )
{
System.out.println( "Syntax: java RSA PrimeSize" ) ;
System.out.println( "e.g. java RSA 8" ) ;
System.out.println( "e.g. java RSA 512" ) ;
System.exit( -1 ) ;
}
// Get bit length of each prime number
int primeSize = Integer.parseInt( args[0] ) ;
// Generate Public and Private Keys
RSA rsa = new RSA( primeSize ) ;
System.out.println( "Key Size: [" + primeSize + "]" );
System.out.println( "" ) ;
System.out.println( "Generated prime numbers p and q" );
System.out.println( "p: [" + rsa.getp().toString( 16 ).toUpperCase() + "]" );
System.out.println( "q: [" + rsa.getq().toString( 16 ).toUpperCase() + "]" );
System.out.println( "" ) ;
System.out.println( "The public key is the pair (N, E) which will be published." ) ;
System.out.println( "N: [" + rsa.getN().toString( 16 ).toUpperCase() + "]" ) ;
System.out.println( "E: [" + rsa.getE().toString( 16 ).toUpperCase() + "]" ) ;
System.out.println( "" ) ;
System.out.println( "The private key is the pair (N, D) which will be kept private." ) ;
System.out.println( "N: [" + rsa.getN().toString( 16 ).toUpperCase() + "]" ) ;
System.out.println( "D: [" + rsa.getD().toString( 16 ).toUpperCase() + "]" ) ;
System.out.println( "" ) ;
// Get message (plaintext) from user
System.out.println( "Please enter message (plaintext):" ) ;
String plaintext = ( new BufferedReader( new InputStreamReader( System.in ) ) ).readLine() ;
System.out.println( "" ) ;
// Encrypt Message
BigInteger[] ciphertext = rsa.encrypt( plaintext ) ;
System.out.print( "Ciphertext: [" ) ;
for( int i = 0 ; i < ciphertext.length ; i++ )
{
System.out.print( ciphertext[i].toString( 16 ).toUpperCase() ) ;
if( i != ciphertext.length - 1 )
System.out.print( " " ) ;
}
System.out.println( "]" ) ;
System.out.println( "" ) ;
String recoveredPlaintext = rsa.decrypt( ciphertext ,rsa.getD(),rsa.getN()) ;
System.out.println( "Recovered plaintext: [" + recoveredPlaintext + "]" ) ;
}
}
/*ServerForm*/import javax.swing.*;
import java.util.*;
import java.net.*;
import java.io.*;
import java.sql.*;
import java.math.*;
public class ServerForm extends javax.swing.JFrame {
String key_result="";
public ServerForm() {
initComponents();
userInitComponents();
}
public void userInitComponents(){
setBounds(0,0,800,600);
}
private void initComponents() {//GEN-BEGIN:initComponents
p_container = new javax.swing.JPanel();
p_box = new javax.swing.JPanel();
b_start_server = new javax.swing.JButton();
b_unstegno = new javax.swing.JButton();
getContentPane().setLayout(null);
setTitle("Server");
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
exitForm(evt);
}
});
p_container.setLayout(null);
p_container.setBackground(new java.awt.Color(153, 153, 255));
p_container.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
p_box.setLayout(null);
p_box.setBackground(new java.awt.Color(130, 102, 50));
p_box.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
b_start_server.setBackground(new java.awt.Color(90, 49, 34));
b_start_server.setFont(new java.awt.Font("Times New Roman", 1, 18));
b_start_server.setForeground(new java.awt.Color(43, 147, 53));
b_start_server.setText("Start Server");
b_start_server.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
b_start_server.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
b_start_serverActionPerformed(evt);
}
});
p_box.add(b_start_server);
b_start_server.setBounds(150, 30, 120, 30);
b_unstegno.setBackground(new java.awt.Color(90, 49, 34));
b_unstegno.setFont(new java.awt.Font("Times New Roman", 1, 18));
b_unstegno.setForeground(new java.awt.Color(255, 255, 255));
b_unstegno.setText("UnStegno");
b_unstegno.setBorder(new javax.swing.border.BevelBorder(javax.swing.border.BevelBorder.RAISED));
b_unstegno.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
b_unstegnoActionPerformed(evt);
}
});
// p_box.add(b_unstegno);
b_unstegno.setBounds(350, 30, 130, 30);
p_container.add(p_box);
p_box.setBounds(100, 380, 620, 80);
getContentPane().add(p_container);
p_container.setBounds(0, 0, 800, 600);
pack();
}//GEN-END:initComponents
private void b_unstegnoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_b_unstegnoActionPerformed
hide();
}//GEN-LAST:event_b_unstegnoActionPerformed
private void b_start_serverActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_b_start_serverActionPerformed
BigInteger n = null;
String pass=null;
BigInteger d = null;
BigInteger [] enc = null;
String dhash=null;
String rhash=null;
Vector recived_packet=new Vector();
Vector send_packet=new Vector();
Socket server=null;
boolean serverstart=true;
try{
ServerSocket serversocket=new ServerSocket(4444);
System.out.println("Server Listening...");
System.out.println("Server Listening......");
while(serverstart){
server=serversocket.accept();
System.out.println("Server accept...");
ObjectInputStream fromclient=new ObjectInputStream(server.getInputStream());
recived_packet=(Vector)fromclient.readObject();
String header=(String)recived_packet.get(0);
//response based on the header
//Login Process
if(header.equalsIgnoreCase("login")){
System.out.println("Login Data Service");
String id=(String)recived_packet.get(1);
System.out.println("Server reading client data...");
//String pass=(String)recived_packet.get(2);
rhash=(String)recived_packet.elementAt(2);
enc =(BigInteger[]) recived_packet.elementAt(3);
System.out.println("Ciphertext at receiver side"+enc);
StringBuffer bf = new StringBuffer();
for( int i = 0 ; i < enc.length ; i++ ){
bf.append( enc[i].toString( 16 ).toUpperCase() ) ;
if( i != enc.length - 1 )
System.out.print( " " ) ;
}
String message=bf.toString();
//System.out.println("Message"+message);
n = (BigInteger)recived_packet.elementAt(4);
d = (BigInteger)recived_packet.elementAt(5);
//in.close();
byte buf[] = message.getBytes();
MD5 md = new MD5();
byte out[] = new byte[16];
md.update(buf);
md.md5final(out);
String nhash = md.dumpBytes(out);
System.out.println("Hashcode"+rhash);
if(rhash.equals(nhash))
{
RSA rsa = new RSA( 8 ) ;
pass = rsa.decrypt( enc ,d,n) ;
System.out.println("Decrypted Message"+message);
//System.out.println("Correct message"+dhash);
}
String result=loginValiddation(id,pass);
send_packet.add(0,result);
}
//Encryption Process
ObjectOutputStream toclient=new ObjectOutputStream(server.getOutputStream());
toclient.writeObject(send_packet);
System.out.println("Server send data to client...");
fromclient.close();
toclient.flush();
toclient.close();
if(!serverstart){
System.out.println("**************************Server Stop*****************************");
}
}
JOptionPane.showMessageDialog(this,"FileRecived From Client :"+server.getInetAddress(),"Server Message",3);
}
catch(Exception e){
e.printStackTrace();
}
}//GEN-LAST:event_b_start_serverActionPerformed
public String loginValiddation(String id,String pass1){
String result="";
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection con=DriverManager.getConnection("jdbc:odbc:serverdsn");
Statement st=con.createStatement();
ResultSet rs=st.executeQuery("select password from clients where userid='"+id+"'");
if(rs.next()){
String pass2=rs.getString(1);
if(pass2.equals(pass1)){
result="valid";
}
else{
result="wrongpass";
}
}
else{
result="invalid";
}
con.close();
}
catch(Exception e){
e.printStackTrace();
}
System.out.println("Server Login Validation Result :"+result);
return result;
}
/* public int storeKeys(String userid,BigInteger privatekey,BigInteger modulus){
int reqnum=0;
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection con=DriverManager.getConnection("jdbc:odbc:serverdsn");
Statement st=con.createStatement();
ResultSet rs=st.executeQuery("select max(reqnum) from clientkeys");
rs.next();
reqnum=rs.getInt(1);
System.out.println("Maxmimum Request Number :"+reqnum);
Statement st1=con.createStatement();
reqnum=reqnum+1;
System.out.println("New Request Number :"+reqnum);
int x=st1.executeUpdate("insert into clientkeys values("+reqnum+",'"+userid+"',"+privatekey.intValue()+","+modulus.intValue()+")");
if(x==1){
key_result="done";
}
else{
key_result="problem";
}
con.close();
System.out.println("New Keys Stored in Database");
}
catch(Exception e){
e.printStackTrace();
}
return reqnum;
}*/
private void exitForm(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_exitForm
System.exit(0);
}//GEN-LAST:event_exitForm
public static void main(String args[]) {
new ServerForm().show();
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton b_start_server;
private javax.swing.JPanel p_box;
private javax.swing.JPanel p_container;
private javax.swing.JButton b_unstegno;
// End of variables declaration//GEN-END:variables
}
Sample ScreenShot:
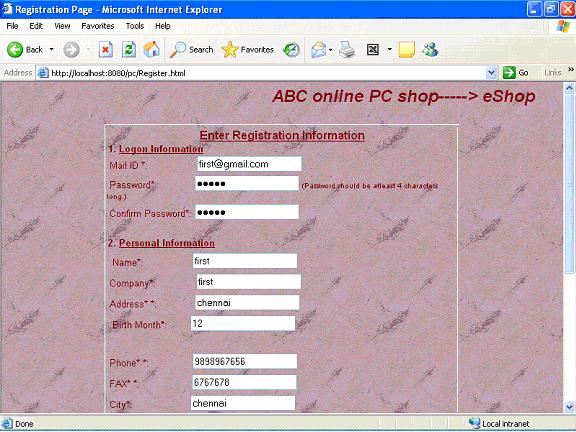
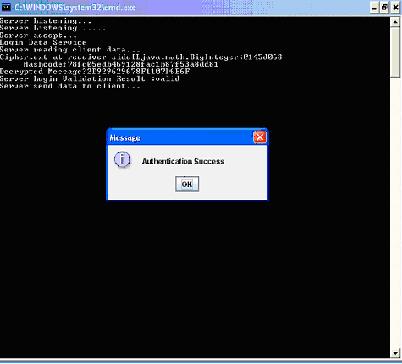
|