|
Java Program To Implement User Interface Components |
Algorithm Steps:
Step 1: Import all necessary packages and classes
Step 2: Define a class that extends frame and implements action listener
Step 3: Declare two text fields and a text area then declare labels for them
Step 4: Declare a button
Step 5: In the class constructor do the following:
i) Create the text fields, text area, and the labels
ii) Set the layout of the frame to border layout
iii) Add the controls to the frame
iv) Add action listener to the button
v) Add window listener to the frame which listens to a window adapter object
Step 6: In the actionPerformed() method, add the text of all the text fields to the text area
Step 7: In the main method () create a frame, set its size and the make it visible
Step 8: Define a Window Adapter class and handle the window closing event
Java Program To Implement User Interface Components
import java.awt.*;
import java.awt.event.*;
class TextFrame extends Frame implements ActionListener
{
TextField txtName;
TextField txtRollNo;
TextArea areaDisplay;
Label lblName,lblRollNo,lblDisplay;
Button btnSubmit,btncancel;
Choice c;
TextFrame()
{
Panel p1=new Panel();
Panel p2=new Panel();
Panel p3=new Panel();
Panel p4=new Panel();
txtName = new TextField(20);
txtRollNo = new TextField(10);
lblRollNo = new Label("Phone Number ");
areaDisplay = new TextArea(5,25);
lblName = new Label("Name ");
btnSubmit = new Button("Submit");
btncancel = new Button("cancel");
lblDisplay = new Label("You Have Entered The Following Details");
c=new Choice();
c.add("java/j2ee");
c.add(".net");
c.add("multimedia");
p1.add(lblName);
p1.add(txtName);
p2.add(lblRollNo);
p2.add(txtRollNo);
p3.add(btnSubmit);
p3.add(btncancel);
p4.add(areaDisplay);
add(p1);
add(p2);
add(c);
add(p3);
add(lblDisplay);
add(p4);
p1.setLayout(new FlowLayout(FlowLayout.LEFT,0,10));
// p2.setLayout(new FlowLayout());
// p3.setLayout(new FlowLayout(FlowLayout.CENTER,200,100));
p4.setLayout(new FlowLayout(FlowLayout.CENTER,0,250));
add(areaDisplay);
setLayout(new FlowLayout(FlowLayout.LEFT,10,10));
btnSubmit.addActionListener(this);
addWindowListener( new MyWindowAdapter(this));
// addWindowListener( new MyWindowAdapter1(this));
}
public void actionPerformed(ActionEvent e)
{
areaDisplay.setText("\n Name: " +txtName.getText()+"\n Roll Number: " + txtRollNo.getText()+" \n course selected is : " + c.getSelectedItem());
}
public static void main(String[] a)
{
TextFrame frame = new TextFrame();
frame.setSize(400,400);
frame.setVisible(true);
}
}
class MyWindowAdapter extends WindowAdapter
{
TextFrame frame;
MyWindowAdapter(TextFrame frame)
{
this.frame=frame;
}
public void windowClosing(WindowEvent evt)
{
frame.dispose();
System.exit(0);
}
}
SAMPLE OUTPUT SCREEN:
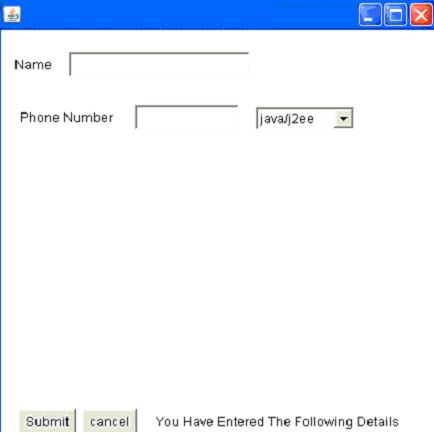
|
|
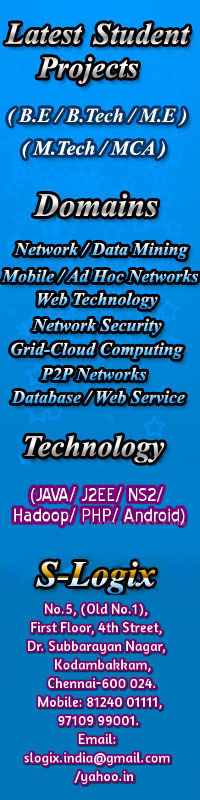
⇓Student Projects⇓
⇑Student Projects⇑
|