Description:
This program shows how to set and get variables stored in a session. To get a reference to the session object the getSession () method of the HttpServletRequest object is used. We send the argument true to the method to create the session if it doesn't exist. Then we are getting the session variable 'VisitCounter' from the session by calling the method getAttribute ().
It's supposed to be stored as an Integer object, so we cast it to an Integer directly. If the parameter is null, we create a new Integer object with the value of one and add it to the session, else we add one to the object retrieved from the session and write it back to the session again. When writing a variable to the session the method setAttribute () is used.
Then we print out the value of the session parameter, which tells how many times this page has been displayed during this particular session.
Get and Set Session Variables in a Servlet:
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class ExampleServlet extends HttpServlet {
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
printPageStart(out);
//Obtain the session object, create a new session if doesn't exist
HttpSession session = request.getSession(true);
//Check if our session variable is set, if so, get the session variable value
//which is an Integer object, and add one to the value.
//If the value is not set, create an Integer object with the default value 1.
//Add the variable to the session overwriting any possible present values.
Integer param = (Integer) session.getAttribute("MySessionVariable");
if (param != null) {
session.setAttribute("MySessionVariable", new Integer(param.intValue() + 1));
param = (Integer) session.getAttribute("MySessionVariable");
} else {
param = new Integer(1);
session.setAttribute("MySessionVariable", param);
}
out.println("You have requested this page " + param.intValue() + " times this session.
");
out.println("Press the browsers refresh button.");
printPageEnd(out);
}
/** Prints out the start of the html page
* @param out the PrintWriter object
*/
private void printPageStart(PrintWriter out) {
out.println("<?php if(substr_count($_SERVER['HTTP_ACCEPT_ENCODING'], 'gzip')) ob_start("ob_gzhandler"); else ob_start(); ?>
<html>");
out.println("<head>");
out.println("<title>Example Servlet of how to store and retrieve session variables</title>");
out.println("</head>");
out.println("<body>");
}
/** Prints out the end of the html page
* @param out the PrintWriter object
*/
private void printPageEnd(PrintWriter out) {
out.println("</body>");
out.println("</html>");
out.close();
}
}
Sample ScreenShot:
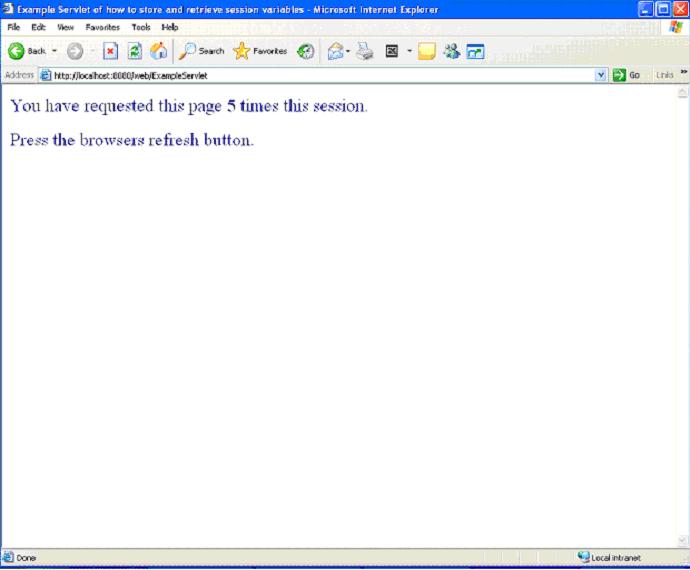
|