We can make text files in every editor, notepad is preferably used to make text files. The extension of the text file is .txt . Lets first make a simple interface that lets us enter some data in the multi-line textbox. Reading is also as simple as writing the text files.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace filestream
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
FileStream fs = File.Create("Test.txt");
StreamWriter sw = new StreamWriter(fs);
sw.Write(textBox1.Text);
sw.Close();
fs.Close();
}
private void button2_Click(object sender, EventArgs e)
{
FileStream fs = File.OpenRead("Test.txt");
StreamReader sr = new StreamReader(fs);
while (sr.Peek() > -1)
{
MessageBox.Show(sr.ReadLine());
}
sr.Close();
fs.Close();
}
}
}
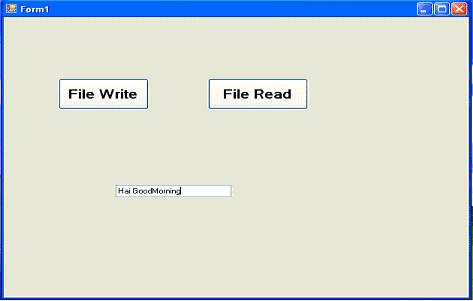
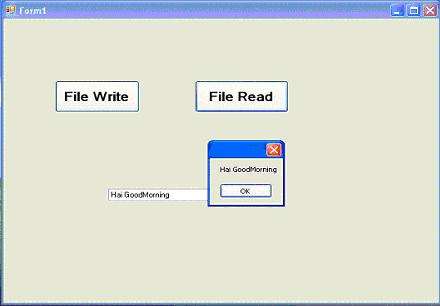
|