For files with known internal structures, the BinaryReader and BinaryWriter classes offer streaming functionality that's oriented towards particular data types. The good thing about writing binary files is you cannot read the files by just opening them in the notepad also binary files can be of very large size.
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace filestream
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
FileStream fs = File.Create("picture.dat");
BinaryWriter bw = new BinaryWriter(fs);
int x = 10;
decimal d = 3.234M;
string str = "Hello World";
bw.Write(x);
bw.Write(d);
bw.Write(str);
bw.Close();
fs.Close();
}
private void button2_Click(object sender, EventArgs e)
{
FileStream fs = File.OpenRead("picture.dat");
BinaryReader br = new BinaryReader(fs);
label1.Text = System.Convert.ToString(br.ReadInt32());
label2.Text = System.Convert.ToString(br.ReadDecimal());
MessageBox.Show(br.ReadString());
br.Close();
fs.Close();
}
}
}
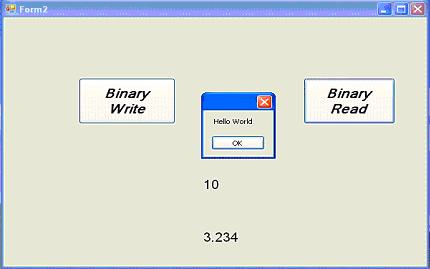
|